PhpOffice/PhpWord
。这个库提供了丰富的API来处理Word文档。在现代Web开发中,PHP作为服务端脚本语言被广泛应用,有时候我们需要处理Word文档,比如读取其内容、提取信息等,本文将详细介绍如何使用PHP读取Word文档的内容。
使用PHP读取Word文档
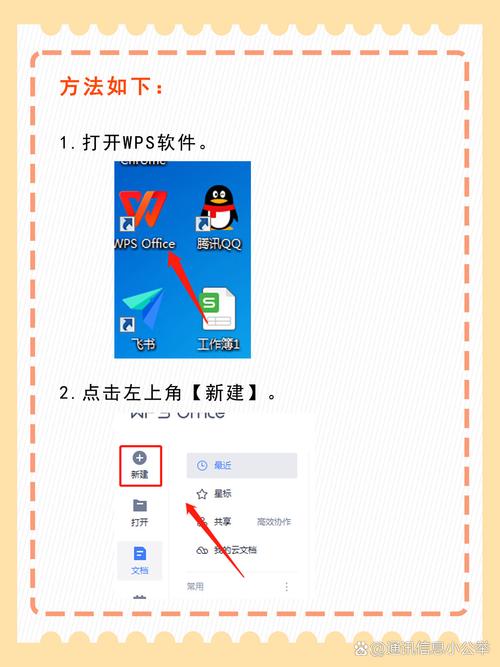
方法一:使用phpword
库
1、安装phpword
库
要使用phpword
库来读取Word文档,首先需要安装该库,可以通过Composer来管理依赖:
```bash
composer require phpoffice/phpword
```
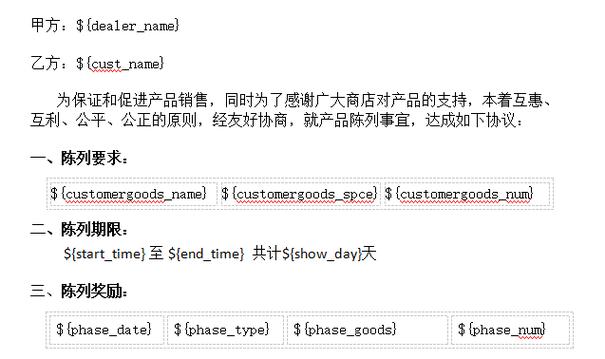
2、读取Word文档
安装好库后,我们可以编写代码来读取Word文档,以下是一个简单的示例:
```php
<?php
require 'vendor/autoload.php';
use PhpOffice\PhpWord\IOFactory;
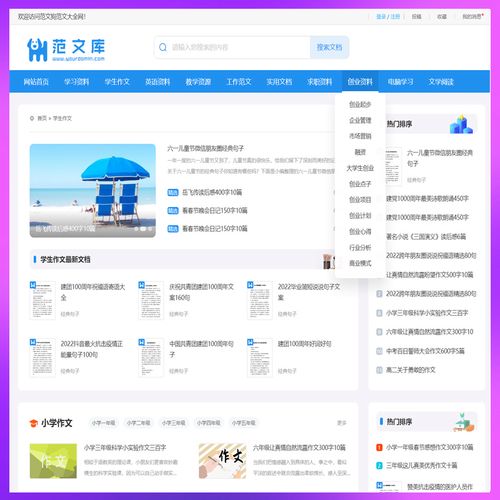
// 加载Word文档
$file = 'path/to/your/document.docx';
$phpWord = IOFactory::load($file);
// 遍历文档中的段落并输出
foreach ($phpWord->getSections() as $section) {
foreach ($section->getElements() as $element) {
if (method_exists($element, 'getText')) {
echo $element->getText() . "
";
}
}
}
?>
```
方法二:使用PHPWord
和XMLReader
如果你不想使用第三方库,也可以通过解析Word文档的XML结构来读取内容,这种方法较为复杂,但可以不依赖外部库。
1、打开Word文档
需要将Word文档解压并读取其中的XML文件,Word文档实际上是一个压缩包,可以使用ZipArchive类来解压。
```php
<?php
$zip = new ZipArchive;
if ($zip->open('path/to/your/document.docx') === TRUE) {
$xmlFile = 'word/document.xml';
if (($index = $zip->locateName($xmlFile)) !== FALSE) {
$text = $zip->getFromIndex($index);
$xml = simplexml_load_string($text, 'SimpleXMLElement', LIBXML_NOCDATA);
}
$zip->close();
} else {
echo 'Failed to open the document';
}
?>
```
2、解析XML并提取文本
通过遍历XML节点来提取文本内容。
```php
<?php
function extractText($node) {
$text = '';
foreach ($node->children() as $child) {
if (!empty($child->text())) {
$text .= $child->text() . "
";
}
$text .= extractText($child);
}
return $text;
}
$text = extractText($xml);
echo $text;
?>
```
方法三:使用antiword
工具
antiword
是一个命令行工具,可以将Word文档转换为纯文本,你可以通过PHP执行系统命令来调用它。
1、安装antiword
你需要先在你的系统中安装antiword
,在Debian/Ubuntu上,你可以使用以下命令安装:
```bash
sudo apt-get install antiword
```
2、使用PHP执行系统命令
你可以在PHP中使用exec
函数来调用antiword
并获取输出。
```php
<?php
$file = 'path/to/your/document.doc'; // 确保文件是.doc格式,而不是.docx
$output = shell_exec("antiword " . escapeshellarg($file));
echo $output;
?>
```
相关问答FAQs
Q1: 如何确保从Word文档中提取的文本格式正确?
A1: 由于Word文档可能包含复杂的格式和嵌入对象,提取的文本可能需要进一步处理以去除多余的空格、换行符或格式化字符,可以使用正则表达式或字符串操作函数来进行清理,使用 Q2: 如果Word文档非常大,读取时性能不佳怎么办? A2: 对于大型文档,直接读取可能会消耗大量内存和时间,可以考虑以下优化策略:逐段读取文档内容而不是一次性加载整个文档;或者使用流式处理方式,只处理需要的部分,也可以考虑使用更高效的文本处理算法或数据结构来提高性能。 以上就是关于“php怎么读取word文档”的问题,朋友们可以点击主页了解更多内容,希望可以够帮助大家!trim()
去除首尾空白,使用nl2br()
将换行符转换为HTML的<br>